One of the recurring problems I have at my job is deployment. What I mean is getting the code from development environment to production environment. One of the most interesting tasks is how to deploy php application on server.
In this article, I want to show you how to push your site to remote host using various modern deployment tools (which will go much further than simple FTP).
Deploy repo w/o dependencies and artifacts and build your app on the server
- First, we need to build and test the app. We can do it either locally, on a build server (eg. Jenkins), or in cloud (Buddy).
- Once the tests pass, we can upload the source code to the server (in this case without dependencies and artifacts) via FTP, SFTP or Rsync.
- The final step is downloading the dependencies and building the app on the server. The easiest way is to run a script on the server via SSH.
The whole process looks like this:
PHP Delivery Workflow #1
Pros & Cons
- The build environment of the app is exactly the same as the running environment.
- Dependencies will download faster as they’re fetched from the closest mirror.
- If you don’t use any mechanism to minimize the downtime (eg. atomic deployment), the time required to download the dependencies and build the application may extend it.
- The build time may be long and impact the performance of the production server
Deploy repo with dependencies and artifacts
In this workflow, the application is first compiled and tested, then deployed to the server in its final form (with dependencies and artifacts):
PHP Delivery Workflow #2
Pros & Cons
- The production server is not stressed with the build.
- The application on the production server is the same as the application from the test server.
- You don’t need to SSH to the server to run scripts = any old school FTP will do.
- You must provide the same build environment as the running environment for the application.
- Since we deploy everything (including dependencies), the upload time can be long.
GitHub
If you are a developer, then you must know about Git for source code management. Developers use GitHub when it comes to interaction with multiple team members and open source contributors for developing coding solutions. I’ve written a short series on Git for beginners covering, commands cheat sheets, Branches, Conflicts etc.
The best thing about Git is that it allows developers to create custom workflows manually, or by integrating third party PHP deployment tools.
Cloudways allows you to deploy code of your application from your Git repositories. Your Git repository must support Git over SSH for this to work. For Git deployment, you must follow simple steps given below.
Signup & Launch Server
First of all, signup at Cloudways and launch your server and application. Next, move to the Application tab by selecting any app from application page.

Generating SSH Keys
Here, you must download SSH keys by moving to Deployment via Git tab,
We will use these keys to allow access from your Cloudways server to your git repository. Now click on the Generate SSH Keys button to generate the keys.

Now, click on Download SSH Keys to download SSH Public Key that we will use in the next step.

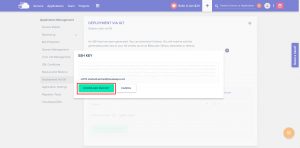
Upload SSH Key To GitHub Repository
On Github, navigate to the repository and find the code which you want to deploy. If you are using another Git service, you will have to find the equivalent way of deploying them. Go to Settings -> Deploy keys and click on the Add Deploy Key button to add the SSH key. You can also give a name to this key in the title field and copy the key to the box. Click on the Add Key button to save the SSH key.

Copy the SSH Address Of Repository
Copy the repository address as shown in the image below. Make sure to copy the SSH address as other formats (like HTTPS) are not supported.

Deploy Code from Your Repository
- Go back to Cloudways console. Paste the SSH address you got in Step 4 into the Git Remote Address” field.
- Select the branch of your repository you want to deploy from. In this example, we are using the master ” branch.
- Type the deployment path (i.e. the folder in your server where the code will be deployed). Make sure to end it with a backslash (/). If you will leave this field empty, the code will be deployed to public_html/.
- Click on the Start Deployment button to deploy your code to the selected path.
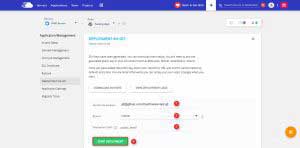
Repository Successfully Cloned
You will get a notification once the deployment process finishes.
You have further options to delete the repository from the server (no files will be deleted, see FAQ below). Pull the latest changes or change the branch you deploy from.

Zero-downtime / Atomic deployment
The workflows above have one flaw: the downtime. This means your application will not be available for the client during the deployment. The solution to that is very simple: deploy and build the application in a different folder than the one from which it’s served.
Atomic deployment
The process involves creating a couple of directories on the server:
/current
- a symbolic link to the current version in the releases directory to which your web server points.
/releases
- contains the history of uploaded. For each version a directory with the name of the revision tag is created.
/deploy-cache
- used for storing new files during the deployment. Once the upload has finished, its contents are copied to a new directory in the
/releases
directory.
- used for storing new files during the deployment. Once the upload has finished, its contents are copied to a new directory in the
Here’s how it works:
- A new version of the application is uploaded to
deploy-cache
- The contents of
deploy-cache
are copied to/releases/${revision}
- The current symbolic link is switched to
/releases/${revision}
Atomic Deployment Template
Pros & Cons
- Downtime reduced basically to zero (the time required to create a symlink)
- Instant rollback – the previous version of application remains on the server after the deployment; all you need to do is switch back the symlink
- More space on the server is required to keep the previous revisions
- A lot of scripts to write (although it’s a one-time job)
The Git variation
This is basically the same as #1, but requires Git installed on the production server. The deployment is made with git push
instead of regular file upload. Then, in Git, the post-receive hook triggers the build.
This method has been very well covered in this article.
PHP Delivery Workflow #2
Pros & Cons
- Since this method employs Git mechanisms, the deploy is faster because only changesets are deployed
- You don’t need to run SSH scripts, because the webhook will call them on the server
- If you don’t use any mechanism to minimize the downtime (eg. atomic deployment), the time required to download the dependencies and build the application may extend the downtime
- The build time may be long and impact the performance of the production server
Docker deployment
Docker is a virtualization method that allows you to define the working environment of your application in a single text file (Dockerfile), together with the way it should be built. The file is then used to build a Docker image with your app that can be launched in any environment supporting Docker (Linux/Mac OS/Windows). Docker is very fast and lightweight, contrary to “traditional” virtualization methods.
Here’s an example Dockerfile for a PHP application:
FROM php:7
RUN apt-get update -y && apt-get install -y openssl zip unzip git
RUN curl -sS https://getcomposer.org/installer | php -- --install-dir=/usr/local/bin --filename=composer
RUN docker-php-ext-install pdo mbstring
WORKDIR /app
COPY . /app
RUN composer install
CMD php artisan serve --host=0.0.0.0 --port=8181
EXPOSE 8181
The file can be used to build a Docker image:
docker build -t my-image .
Once the image is built, you can launch your application in a Docker container isolated from its host
docker run -p 8081:8081 my-image
On top of that, Docker images can be pushed and pulled from a Docker registry. This way you can easily build and image on one server/PC and run it on another. In this case, the whole delivery process will look like this:
- Test application
- Build Docker image with application
- Push application to Docker registry
- SSH to production server a. Docker pull b. Docker run
Atomic Deployment Template
Pros & Cons
- The application works on every type of setup which eliminates the “strange, it works for me” error
- Easy rollback – you just run the previous version of the image
- Build configuration and application environment is documentation in the Dockerfile in the repository
- Yet another tech that adds to the software stack
- Some users claim that Docker is not production ready
DeployHQ
DeployHQ is an amazing PHP deployment tool to automate your deployments from Git, Mercurial, and Subversion code repositories. Git-based deployment is now becoming de-facto standard in any good development agency. This makes lives easy as it reduces the hassle of uploading and downloading source files.
In this post, I will describe how you can integrate DeployHQ with your web app that you have hosted on Cloudways. This integration will ensure on-the-go deployment of the code. With Cloudways staging-friendly environment, developers can experiment with their code as much as they want.
So, here are the steps.
Create a DeployHQ account
Register an account on DeployHQ. (You can use this free account for one project and 10 deployments per day)
Create a new project in DeployHQ
You will need to create a project to start your deployment process.


Connect DeployHQ with your code repository
Enter the details of your code repository (or “code repo”). DeployHQ has out-of-the-box support for popular code hosting sites, like Github, Bitbucket, etc.
Add Path Of Github Repository
To begin with deployment, you need to add the repository path from GitHub like this.
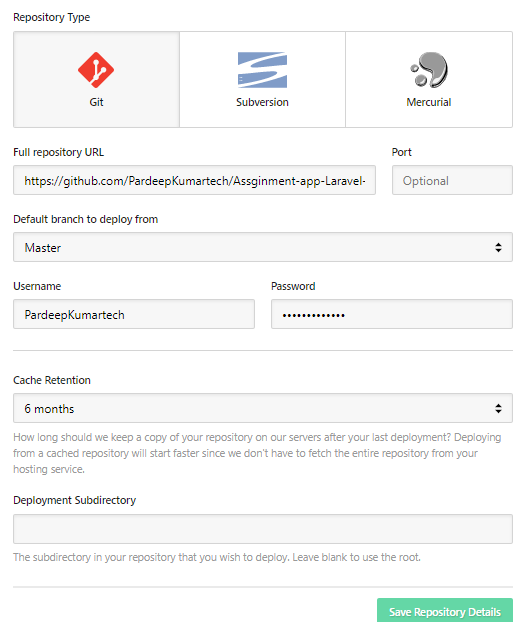
Configure the server
Select SSH/SFTP as protocol
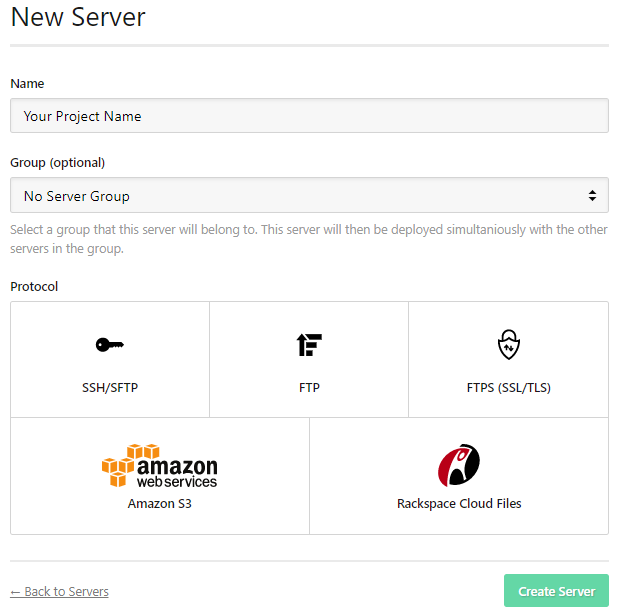
Then, fill up the SSH Configuration.

For example, you may need to change the branch from master to any other branch that you like to deploy from.
Now, click “Save”. You have successfully configured your server
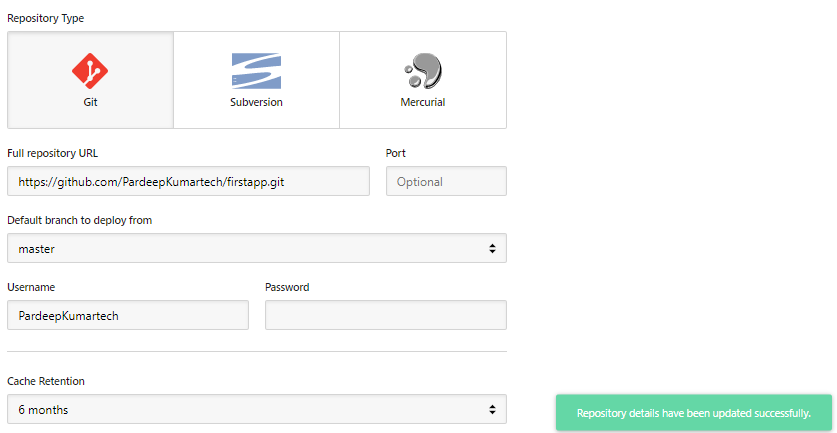
Deploy
Click “Deploy Now”. On the deployment screen, you can click the “Deploy” button to start the deployment process instantly.
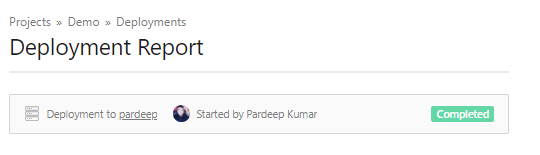

Conclusion
Deploying PHP applications has never been made easier, and you probably never thought that one day you’d be able to use a GUI to set up a php webserver. Well if you are reading this blog post, chances are that deploying your PHP application hasn’t been easier.